
In this chapter we are gonna try a new electronic component: buzzer, a audio signaling device. We will use it to make an alarm device. The buzzer generates sounds at different frequencies when driven by sine wave signals. Combine a LED with the same sine wave, then we can make an alarm device.

Connect the positive of the buzzer to digital pin 8, negative to GND.


Example Program 6-1:

Download the programs into Mind+, then you will hear that the buzzer buzzes a low pitch and a high pitch alternatively, just like a car alarm.

Code Learning 1
1.#include <DFRobot_Libraries.h>
2.// Create an object
3.DFRobot_Tone DFTone;
4.
5.// Main program starts
6.void setup() {
7.
8.}
9.void loop() {
10. DFTone.play(8, 131, 250);
11. DFTone.play(8, 523, 250);
12.}
There is only one new function in the loop: DFTone.Play().
Block in Mind+:

The format of the function:

If you want to use buzzer to make more various sounds, then graphical programming Mind+ may cannot meet your requirements since there are limited options of beat in the block(1=1 second).

Although the graphical blocks in Mind+ are easy to use, the flexibility of the program is greatly reduced to a certain extent. So now, let’s get rid of the graphical programming and complete the following project only by coding.
Programming
Input the example program 6-2 into Mind+:
//Project 6 Alarm Device
float sinVal;
int toneVal;
#include <DFRobot_Libraries.h>
// Create an object
DFRobot_Tone DFTone;
void setup(){
pinMode(8, OUTPUT);
}
void loop(){
for(int x=0; x<180; x++){
//Convert the degree of sin function into radian
sinVal = (sin(x*(3.1415/180)));
//Use sin function to generate the sound frequency
toneVal = 2000+(int(sinVal*1000));
//A signal for Pin 8 buzzer to output
DFTone.play(8, toneVal,2);
}
}
Once you have uploaded the code, the buzzer will start to emit sounds with pitches from low to high, and then high to low. Unlike the graphical block, coding makes the output result more flexible.
Code Learning 2
First of all, define two variables:
float sinVal;
int toneVal;
As we mentioned in project 3, the “float” is a data type that allows a variable to store decimal values. The sinVal float variable will hold the sine value that will cause the tone to rise and fall. The toneVal variable will be used to take the value in sinVal and convert it to a frequency we require.
#include
We will go into details about library and object in Chapter 10. It will be much easier to understand after having been used several times.
void setup(){
pinMode(8, OUTPUT);
}
There is function pinMode() in the setup function, and its format as follows:

The pinMode function is used to configure a specific pin to behave either as an input or an output. We may not find this function in Mind+ since it has been encapsulated in other commands such as digitalWrite(pin).
We use the function sin() to calculate the sine value of an angle. Unit: rad. To prevent the function outputting negative number, the range of the for loop is set to 0~179, which is 0~180°.
for(int x=0; x


Buzzer
A buzzer is an electronic component that generates sound. There are generally two types: piezo buzzer and magnetic buzzer.
The difference of piezo buzzer and magnetic buzzer
A piezo buzzer generates sound because of the piezoelectric effect from the piezoelectric ceramic which drives the metallic diaphragm to vibrate. Normally, we have to provide voltage over 9V to have enough SPL. On the other hand, a magnetic buzzer can be driven to generate 85 dB by only 1.5V, but the consumption of the current will be much higher than the Piezo one.
According to the difference of control method, we can divide buzzer into active type and passive type.
The difference between active buzzer and passive buzzer
The active buzzer has an internal oscillating source, and the buzzer will sound as soon as it is energized. While the passive buzzer does not have internal oscillating source, and it has to be driven with square wave and different frequency needed. It is like an electromagnetic changing input signal produces the sound, rather than producing a tone automatically.
In terms of appearance, the active buzzer has a long lead(positive) and a short lead(negative), and the passive buzzer only has two same leads.
In the kit package, we select the magnetic passive buzzer that is suitable for beginners. If you are interested in active buzzer, get one and try it!
Buzzers are widely used in various applications, for instance, infrared sensors and ultrasonic sensors for monitoring object approaching and altering people, gas sensors for gas leaking alarm, or using them as musical instruments to play notes. So amazing, right!

1. Input the codes of this project into Mind+ manually.
2. Combine with an LED to make an alarm device. Tip: use the sin function to make the sound and lighting keep the same frequency.
3. Use the button we introduced in chapter 3 to make a doorbell. When the button is pressed, the buzzer makes a sound.
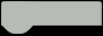